What is JavaScript ?
What is javascript, How to use javascript in web and app, Why to use javascript, different applications of javascript, Libraries and framework of javascript
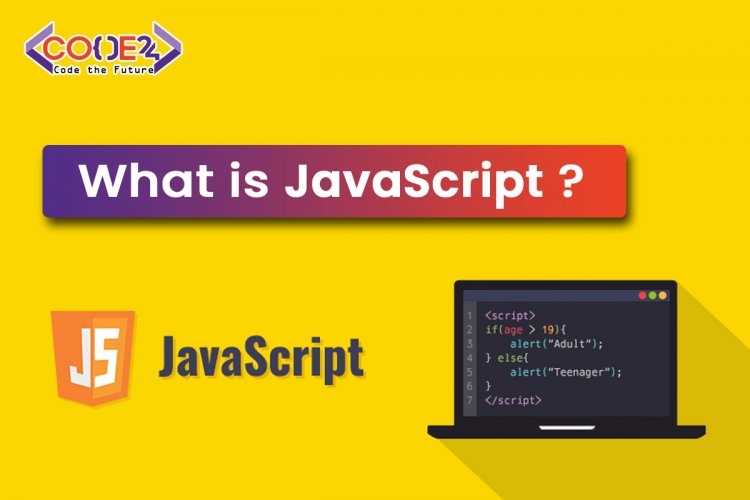
In technology, you can't get very far without running into JavaScript.You probably are here right now because you have heard the term and are aware that you need to comprehend it. There is good news: You can learn to use JavaScript, and it isn't as scary as it sounds.
JavaScript: A Quick Definition
A scripting language called JavaScript is used to create and control dynamic website content, which is anything that moves, refreshes, or otherwise changes on your screen without you having to reload a web page. Examples include:
- animated graphics
- photo slideshows
- autocomplete text suggestions
- interactive forms
Think about web features you use every day and probably take for granted, like when your Facebook timeline updates automatically on your screen or when Google suggests search terms based on a few letters you've started typing. This is an even better way to comprehend what JavaScript does. That is JavaScript in action in both instances.
Although the outcomes of JavaScript may appear straightforward, there is a reason why we cover JavaScript in depth in both our Front End Web Developer and Break into Tech Blueprints courses. There is some pretty fascinating activity taking place beneath all of those fantastic animations and autocompletes. The best ways to learn JavaScript if you realize you need it in your skillset will be explained in detail in this guide, along with how and why to use it.
Table of Contents
- What Is JavaScript? The Basics
- What is JavaScript Used For?
- How Does JavaScript Work on a Site?
- What is Vanilla JavaScript?
- Advanced JavaScript: Libraries and Frameworks
- How to Add JavaScript to a Website
- How to Learn JavaScript?
1. JavaScript: What Is It ? A Comprehensive Explanation
If you want to start a career in technology, your question might be more like this: Is JavaScript required, and what is it?
The answer is a resounding yes if you're interested in web development. With that out of the way, let's examine JavaScript in greater detail.
JavaScript, HTML, CSS
The majority of people begin their coding education with basic HTML and CSS. They move on to JavaScript from there. Which is logical! The foundation of web development is made up of all three components.
In case you are unfamiliar:
HTML is your page's structure—the text in the body, the headers, and any images you want to add.
CSS is what you'll use to customize fonts, background colors, and other aspects of that page's appearance.
The magic third element is JavaScript.JavaScript makes your website or project dynamic after you've created your structure (HTML) and aesthetic vibe (CSS).
Automating and animating with JavaScript
JavaScript is referred to as a "scripting language," as I stated earlier. Coding languages called scripting languages are used to automate steps that users would normally have to take on their own. Any changes made to web pages you visit without scripting would necessitate either manually reloading the page or navigating a series of static menus to reach the content you want.
By instructing computer programs, such as websites or web applications, to "do something," a scripting language like JavaScript (JS, for those who are familiar with it) handles the bulk of the work. This refers to instructing the previously mentioned dynamic features, such as instructing images to animate themselves, photos to cycle through a slideshow, or autocomplete suggestions to respond to prompts, in the context of JavaScript.The "script" in JavaScript is what makes these things appear to happen by themselves.
Meanwhile, all major web browsers include engines that can render JavaScript because JavaScript is so essential to web functionality. Web browsers will be able to comprehend JS commands that are typed directly into an HTML document as a result. To put it another way, there is no need to download any additional software or compilers in order to use JavaScript.
2. What is the Use of JavaScript ?
- This was briefly discussed in the introduction, but here is a quick list of the most common uses of JavaScript.
- Adding interactivity to websites—yes, you'll need to use JavaScript if you want a website to be more than just a page of text.
- Creating mobile applications: JavaScript is used to create apps for smartphones and tablets as well as websites.
- Making games that run in a web browser—Have you ever played a game straight from your browser? That probably happened because of JavaScript.
- Back-end web development: JavaScript is most commonly used on the front end, but it is a scripting language that is sufficiently adaptable to be used on back-end infrastructure as well.
3. How Does JavaScript Work?
JavaScript is either included in a .js file or embedded into a web page. Instead of being a "server-side" language, JavaScript is also a "client-side" language, which means that it is downloaded to the computers of site visitors and processed there.
4. How Do You Add JavaScript to a Website?
The process of actually adding JavaScript code to a web page is fairly straightforward and well-known to anyone who has worked with HTML and CSS. Using the type attribute text/javascript and the <script> tags, JavaScript can be added directly to the code of a page. Sincerely, JavaScript appears to be very comparable to adding CSS to a website. Here is a comparison of the two:
//CSS:
<style>
CSS goes here
</style>
//JavaScript:
<script type="text/javascript">
JavaScript code goes here
</script>
Voila! Additionally, you can add JavaScript code to a page as a separate header file with the extension.js (usually done when you want to include it on multiple pages at once). After that, the script is downloaded and processed by each user's web browser, resulting in the dynamic effects and objects that are displayed on their screens.
However, one word of caution: It is possible for a user to disable JavaScript on their end due to the fact that JavaScript is processed by individual browsers. In the event that this occurs, sites that use JavaScript need to have a backup strategy in place.
5. What is Vanilla JavaScript?
You'll eventually hear the term "vanilla Javascript" as you learn more about JavaScript.What does that imply then?
Vanilla JavaScript is the JavaScript language "as is," with no tools to simplify or speed up the coding process.
Consider the following basic JavaScript code example to get an idea of how vanilla JavaScript looks. If you wanted users to receive a "thanks for signing up" confirmation message after signing up for a service or offer on a website, you would directly code it into an HTML page (between <script> tags) in the following manner:
<script>
window.onload = initAll;
function initAll() {
document.getElementById(“submit”).onclick = submitMessage;
}
function submitMessage() {
var greeting = document.getElementById(“name”).getAttribute(“value”);
document.getElementById(“headline”).innerHTML = “Thank you for joining our email list,” + greeting;
return false;
}
</script>
This kind of vanilla JS can be used to make JavaScript projects. However, as you get more familiar with the JavaScript language, you can use a variety of tools to make using JS easier and more effective.
6. Advanced Javascript: Frameworks and Libraries
Using standard JavaScript on its own, as you might expect, takes forever, and for web developers, time is money. Because of this, your new best friends are JavaScript frameworks and libraries, which are tools that make JS a whole lot easier to use. A breakdown of some of the most common ones and what they do is provided below.
jQuery—The JavaScript Library
When you work with JavaScript, you'll notice JS functions and features like menu animations and fade-outs, file upload forms, and image galleries that are common to multiple websites or web apps. Even though you could code each of these things from the ground up whenever you needed to, using coding libraries like jQuery will make your coding life much simpler.
The JavaScript coding functions that make up the jQuery library are available as single-line jQuery commands.If, for instance, the JavaScript code example above were carried out with jQuery code instead, it would look like this:
<script>
$(“#submit”).click(function () {
var greeting = $(“#name”).val();
$(“#headline”).html(“Thank you for joining our email list, ” + greeting);
return false;
});
<script/>
As you can see, the jQuery programming method is much simpler and can be used whenever you want to code a website or web app with the same JavaScript functionality.
jQuery code can be combined to create more complex plugins in addition to examples like the one above, which are referred to as jQuery snippets—code fragments inserted directly from the jQuery library to carry out specific functions. The jQuery UI (User Interface) repository contains the source code for jQuery plugins, which can be copied and pasted.
React JS—A Front End Developer’s Best Friend
React JS is another important JavaScript library for web developers, along with jQuery.Facebook created the front-end JavaScript library known as React JS in 2011 with the intention of developing user interfaces in mind.UIs are the collection of on-screen menus, search bars, buttons, and anything else a user interacts with in order to use a website or app. You can build UIs manually using vanilla JavaScript, but who has time for that?
For repetitive menu objects and effects like interactive forms, autocomplete features, fade-in or out menu animations, etc., React lets developers use prewritten code. Moreover, a feature known as Virtual DOM, which you can learn more about here, speeds up the site as a whole. As a JavaScript developer, learning how to use React JS will make your life much simpler and increase your employability.
Other JavaScript Libraries and Frameworks
Front-end developers can take things a step further by utilizing tools known as JavaScript frameworks, whereas JS libraries like jQuery and React serve as digital Swiss Army knives for individual coding requirements.
JS frameworks provide JavaScript developers with complete templates for websites or web applications, going beyond jQuery's patchwork functionality. Pre-written code similar to jQuery that can be plugged into those spaces is also created by JS frameworks in those templates where JS code is recommended to be placed.
Even though there are a number of frameworks that are regarded as industry standards, such as Vue and Angular, your best bet when choosing which one to learn is to think about potential clients or employers and determine which JS framework—if any—they prefer. Additionally, keep in mind that picking up additional frameworks is not difficult once you have mastered one.
7. How to Learn JavaScript
The lesson here?JavaScript is the programming language that will bring that page to life, whereas HTML and CSS are the skills that will enable you to code a fundamental web page. Even though learning HTML and CSS will put you in a position to work as a beginning developer, learning JavaScript will help your job prospects grow exponentially.
Our Front End Developer Blueprint is the best place to start if you're looking for a comprehensive JavaScript instructional course.You will learn the ins and outs of JavaScript, the jQuery library, HTML, CSS, and other essential web developer skills in this instructor-led course.
What's Your Reaction?
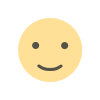
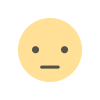
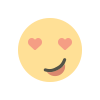
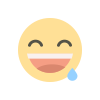
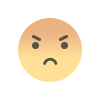
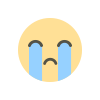
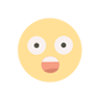