How to upload multiple files using PHP and HTML
upload multiple files using html and php, PHP code to upload multiple files, HTML code to upload multiple file
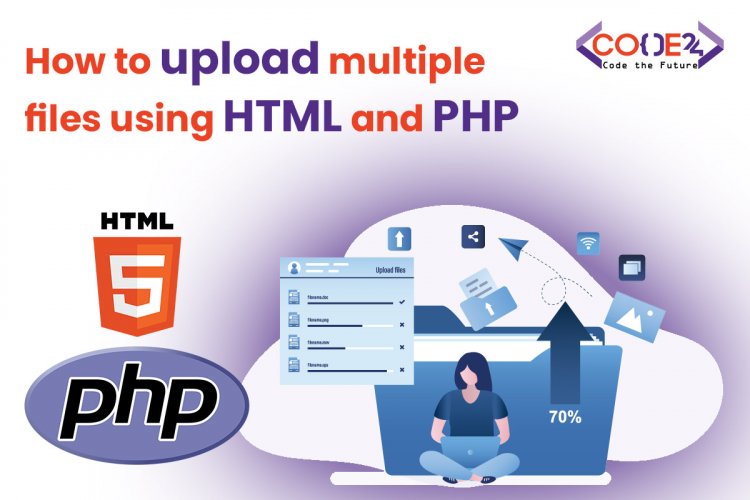
Using a single input file element, you can upload multiple files in PHP. You only need to modify the PHP code for uploading a single file and enable the file element to select multiple files.
We'll look at using HTML and PHP to upload multiple files in this article.The user can select multiple files at once and upload all of them to the server using the multiple image upload feature.
index.html is a file of HTML page that allows users to select multiple files and upload them to the server. A form to select and upload files using the POST method can be found in this HTML file. Which is sending to the server where PHP will handle the rest
<!DOCTYPE html>
<html>
<head>
<title>
Select and upload multiple
files to the server
</title>
</head>
<body>
<!-- multipart/form-data ensures that form data is going to be encoded as MIME data -->
<form action="file_upload.php" method="POST" enctype="multipart/form-data">
<h2>Upload Files</h2>
<p>
Select files to upload:
<!-- name of the input fields are going to
be used in our php script-->
<input type="file" name="files[]" multiple>
<br><br>
<input type="submit" name="submit" value="Upload" >
</p>
</form>
</body>
</html>
in the above code, in <input> section we are giving name as files[ ] where all the selected files will be stored as array. We must add one attribute name multiple in the input tag.
Now to handle the above code in the server, we will make a file with the name file_upload.php in PHP to upload these multiple files to the server.
below is the code of file_upload.php.
<?php
// Check if form was submitted
if(isset($_POST['submit'])) {
// Configure upload directory and allowed file types
$upload_dir = 'uploads'.DIRECTORY_SEPARATOR;
$allowed_types = array('jpg', 'png', 'jpeg', 'gif');
// Define maxsize for files i.e 2MB
$maxsize = 2 * 1024 * 1024;
// Checks if user sent an empty form
if(!empty(array_filter($_FILES['files']['name']))) {
// Loop through each file in files[] array
foreach ($_FILES['files']['tmp_name'] as $key => $value) {
$file_tmpname = $_FILES['files']['tmp_name'][$key];
$file_name = $_FILES['files']['name'][$key];
$file_size = $_FILES['files']['size'][$key];
$file_ext = pathinfo($file_name, PATHINFO_EXTENSION);
// Set upload file path
$filepath = $upload_dir.$file_name;
// Check file type is allowed or not
if(in_array(strtolower($file_ext), $allowed_types)) {
// Verify file size - 2MB max
if ($file_size > $maxsize)
echo "Error: File size is larger than the allowed limit.";
// If file with name already exist then append time in
// front of name of the file to avoid overwriting of file
if(file_exists($filepath)) {
$filepath = $upload_dir.time().$file_name;
if( move_uploaded_file($file_tmpname, $filepath)) {
echo "{$file_name} successfully uploaded <br />";
}
else {
echo "Error uploading {$file_name} <br />";
}
}
else {
if( move_uploaded_file($file_tmpname, $filepath)) {
echo "{$file_name} successfully uploaded <br />";
}
else {
echo "Error uploading {$file_name} <br />";
}
}
}
else {
// If file extension not valid
echo "Error uploading {$file_name} ";
echo "({$file_ext} file type is not allowed)<br / >";
}
}
}
else {
// If no files selected
echo "No files selected.";
}
}
?>
$upload_dir
is used to mention the directory where the image will be saved.
$allowed_types
: it is an array where we can say what kind of file will be supported to store in the server.
$maxsize
: defining the maximum size of the file.
As files are stored in the $_FILES variable. Now we can check whether files are sent from the client side or not using HTML post request with array_filter
function of array in php.
Iterating all the files one by one using the foreach
loop. We will gather information about the files sent. Information such as file name, size, and extensions. We will only allow files that we want to, using
if
(in_array(
strtolower
(
$file_ext
),
$allowed_types
))
this will help filter out all unnecessary files other than the mentioned extensions.
After everything is done we will upload the files in the server using the PHP function move_uploaded_file
.
What's Your Reaction?
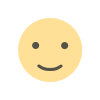
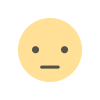
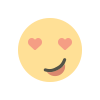
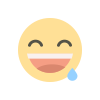
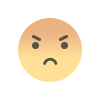
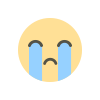
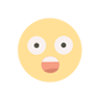