Crush Your PHP Interview with Confidence: 50 Comprehensive Questions
In this comprehensive article, we delved into 50 common interview questions on PHP, providing detailed explanations and answers. Covering a wide spectrum of topics, from basic syntax to object-oriented programming, database interactions, and more, these questions serve as a valuable resource for anyone preparing for a PHP interview. By understanding these concepts and practicing the provided answers, you'll be well-equipped to showcase your knowledge and skills confidently during your interview and increase your chances of success.
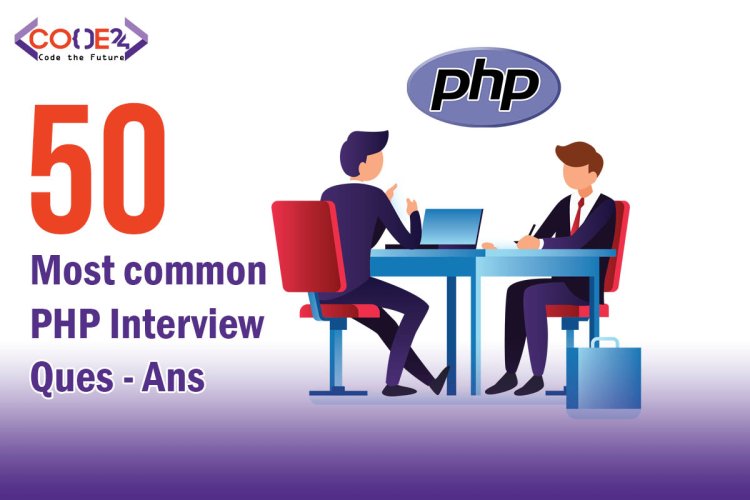
Certainly! Here are the 50 common interview questions on PHP along with their answers:
-
What is PHP and its main use?
- PHP stands for Hypertext Preprocessor, and its main use is to create dynamic web pages and applications.
-
Explain the difference between PHP and HTML.
- HTML is a markup language used to structure content on the web, while PHP is a scripting language used for server-side web development.
-
What is a PHP file extension?
- The file extension for PHP files is ".php".
-
How do you embed PHP code within HTML?
- You can embed PHP code within HTML by using the
tags.
- You can embed PHP code within HTML by using the
-
Describe the characteristics of PHP.
- PHP is a server-side scripting language, open-source, and widely used for web development.
-
What are the types of comments in PHP?
- PHP supports single-line comments (
//
) and multi-line comments (/* */
).
- PHP supports single-line comments (
-
How do you declare a variable in PHP?
- Variables in PHP are declared using the
$
symbol followed by the variable name, like$variableName
.
- Variables in PHP are declared using the
-
Explain the difference between single quotes and double quotes in PHP.
- Single quotes (
'
) treat everything inside as a literal string, while double quotes ("
) allow variables and special characters to be interpreted.
- Single quotes (
-
What is concatenation in PHP?
- Concatenation is combining strings together using the
.
operator.
- Concatenation is combining strings together using the
-
How do you concatenate strings in PHP?
- Strings can be concatenated using the
.
operator, likeecho "Hello, " . $name;
.
- Strings can be concatenated using the
-
What are constants in PHP?
- Constants are values that cannot be changed once defined.
-
How do you define a constant in PHP?
- Constants are defined using the
define()
function, likedefine("PI", 3.14159);
.
- Constants are defined using the
-
What is the difference between == and === in PHP?
==
checks if two values are equal, while===
checks if two values are equal and of the same data type.
-
What is a data type? How many data types are supported in PHP?
- A data type defines the type of value a variable can hold. PHP supports 8 data types, including integers, strings, and arrays.
-
Explain the concept of type juggling in PHP.
- Type juggling is the automatic conversion of data types when performing operations in PHP.
-
What is the ternary operator in PHP?
- The ternary operator (
? :
) is a shorthand way of writing if-else statements.
- The ternary operator (
-
How do you include external files in PHP?
- External files can be included using
include
orrequire
statements.
- External files can be included using
-
Describe the difference between include and require in PHP.
include
will continue execution and generate a warning on failure, whilerequire
will stop execution and generate a fatal error on failure.
-
What is an associative array in PHP?
- An associative array uses named keys instead of numerical indexes.
-
How do you loop through an array in PHP?
- You can use
foreach
loop to iterate through an array.
- You can use
-
Explain the concept of sessions in PHP.
- Sessions are a way to store user data on the server between requests.
-
How do you start a session in PHP?
- Sessions are started using the
session_start()
function.
- Sessions are started using the
-
What is a session variable in PHP?
- A session variable stores user data that can be accessed throughout the session.
-
How do you destroy a session in PHP?
- The
session_destroy()
function is used to end a session and delete session data.
- The
-
Explain the difference between GET and POST methods in PHP.
- Both GET and POST methods are used to send data to the server, but GET appends data to the URL, while POST sends data in the request body.
-
How do you retrieve form data using GET and POST methods?
- For GET, you can use
$_GET['variable_name']
, and for POST, you can use$_POST['variable_name']
.
- For GET, you can use
-
What is the superglobal $_GET array in PHP?
$_GET
is an associative array that holds data sent using the GET method.
-
Explain the use of $_POST and $_REQUEST arrays in PHP.
$_POST
holds data sent using the POST method, while$_REQUEST
holds data from both GET and POST methods.
-
How do you sanitize user input in PHP to prevent SQL injection?
- You can use functions like
mysqli_real_escape_string()
or prepared statements to sanitize user input.
- You can use functions like
-
What is the purpose of the stripslashes() function in PHP?
- The
stripslashes()
function is used to remove backslashes added to escape characters.
- The
-
Explain the use of cookies in PHP.
- Cookies are small pieces of data stored on the client's browser to track user information.
-
How do you set and retrieve cookies in PHP?
- Cookies are set using the
setcookie()
function and retrieved using$_COOKIE
superglobal.
- Cookies are set using the
-
What is the difference between unset() and session_destroy() functions in PHP?
unset()
is used to delete specific session variables, whilesession_destroy()
ends the entire session.
-
Describe the use of include_once and require_once in PHP.
- Both are used to include files, but
include_once
andrequire_once
prevent multiple inclusions of the same file.
- Both are used to include files, but
-
What is the use of error_reporting in PHP?
error_reporting
is used to control the level of error reporting in PHP scripts.
-
Explain the concept of function in PHP.
- A function is a block of code that performs a specific task and can be reused throughout a script.
-
How do you declare and call a function in PHP?
- Functions are declared using
function
keyword and called using their name followed by parentheses.
- Functions are declared using
-
What is a recursive function in PHP?
- A recursive function is a function that calls itself to solve a problem.
-
How do you handle file uploads in PHP?
- File uploads are handled using the
$_FILES
superglobal and themove_uploaded_file()
function.
- File uploads are handled using the
-
Explain the use of file_get_contents() and file_put_contents() functions in PHP.
file_get_contents()
reads the contents of a file, whilefile_put_contents()
writes data to a file.
-
What is the purpose of header() function in PHP?
- The
header()
function is used to send HTTP headers to the client's browser.
- The
-
How do you handle exceptions in PHP?
- Exceptions are handled using the
try
,catch
, and optionallyfinally
blocks.
- Exceptions are handled using the
-
Describe the use of try-catch block in PHP.
- Code within the
try
block is executed, and if an exception occurs, it's caught and handled in thecatch
block.
- Code within the
-
What is object-oriented programming (OOP) in PHP?
- OOP is a programming paradigm that uses objects and classes to structure code.
-
How do you define a class and create an object in PHP?
- A class is defined using the
class
keyword, and objects are created using thenew
keyword.
- A class is defined using the
-
What are access modifiers in PHP OOP?
- Access modifiers (
public
,private
, andprotected
) define the scope of class properties and methods.
- Access modifiers (
-
Explain the concept of inheritance in PHP.
- Inheritance allows a class to inherit properties and methods from another class.
-
What is method overloading and method overriding in PHP OOP?
- Method overloading is having multiple methods with the same name but different parameters. Method overriding is redefining a method in a subclass.
-
How do you use namespaces in PHP?
- Namespaces provide a way to group related classes and avoid naming conflicts.
-
Describe the use of autoloading in PHP.
- Autoloading automatically includes class files when they're needed, improving code organization and efficiency.
These questions cover various aspects of PHP programming and can help you prepare for your PHP interview. Review and practice your answers to stand out during the interview process.
What's Your Reaction?
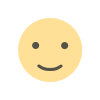
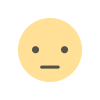
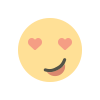
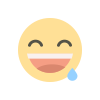
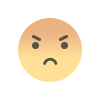
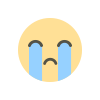
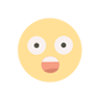