How to resize image and create thumbnail using Codeigniter 3
Codeigniter 3 resize image and create thumbnail example
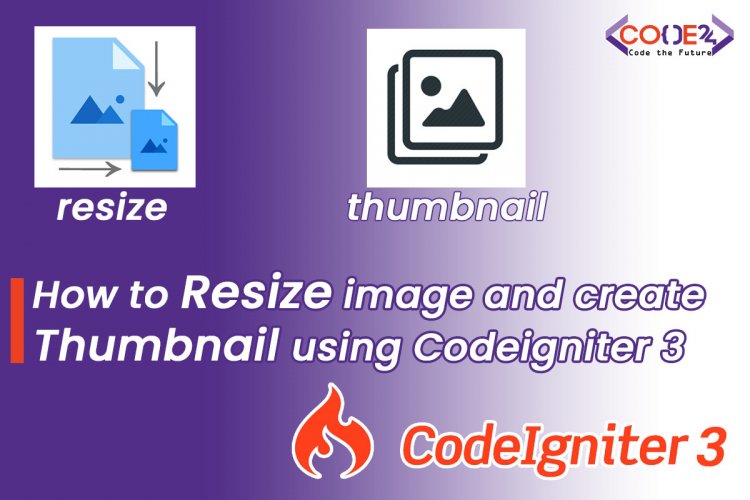
In this post, I'll show you how to use the Codeigniter 3 application to resize an image and generate a thumbnail image after it has been uploaded. In this section, I will demonstrate the application's image upload and resize functionality in detail. In CodeIgniter, we will use the "image_lib" library to resize an image.
In order to reduce the load time, we may occasionally need to generate thumbnail images. If your image is large and only needs to be 100 pixels wide on your listing page, it's best to create a thumbnail image so that our website doesn't take longer to load. Therefore, you can learn from this how to generate thumbnail images in our application if you develop them in CodeIgniter.
You can accomplish this by following a few simple steps; I will demonstrate everything from scratch; simply follow the steps below to view an entire example of image resizing.
Step 1: Download Codeigniter 3
First things first, we need to download the latest version of Codeigniter 3. If you haven't already, you can do so here: Get Codeigniter 3 for free.
Step 2: Create Routes
Here, we will add new routes for image upload. so open routes.php file and add code like as bellow:
application/config/routes.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
$route['default_controller'] = 'welcome';
$route['404_override'] = '';
$route['translate_uri_dashes'] = FALSE;
$route['image-upload'] = 'ImageUpload';
$route['image-upload/post']['post'] = "ImageUpload/uploadImage";
Now, we must create an "ImageUpload" controller with function index(), uploadImage() and resizeImage().Therefore, create the ImageUpload.php file in the following location: application/controllers/ImageUpload.php and insert the following code there:
application/controllers/ImageUpload.php
<?php
class ImageUpload extends CI_Controller {
/**
* Manage __construct
*
* @return Response
*/
public function __construct() {
parent::__construct();
$this->load->helper(array('form', 'url'));
}
/**
* Manage index
*
* @return Response
*/
public function index() {
$this->load->view('imageUploadForm', array('error' => '' ));
}
/**
* Manage uploadImage
*
* @return Response
*/
public function uploadImage() {
$config['upload_path'] = './uploads/';
$config['allowed_types'] = 'gif|jpg|png';
$config['max_size'] = 1024;
$this->load->library('upload', $config);
if ( ! $this->upload->do_upload('image')) {
$error = array('error' => $this->upload->display_errors());
$this->load->view('imageUploadForm', $error);
}else {
$uploadedImage = $this->upload->data();
$this->resizeImage($uploadedImage['file_name']);
print_r('Image Uploaded Successfully.');
exit;
}
}
/**
* Manage uploadImage
*
* @return Response
*/
public function resizeImage($filename)
{
$source_path = $_SERVER['DOCUMENT_ROOT'] . '/uploads/' . $filename;
$target_path = $_SERVER['DOCUMENT_ROOT'] . '/uploads/thumbnail/';
$config_manip = array(
'image_library' => 'gd2',
'source_image' => $source_path,
'new_image' => $target_path,
'maintain_ratio' => TRUE,
'create_thumb' => TRUE,
'thumb_marker' => '_thumb',
'width' => 150,
'height' => 150
);
$this->load->library('image_lib', $config_manip);
if (!$this->image_lib->resize()) {
echo $this->image_lib->display_errors();
}
$this->image_lib->clear();
}
}
?>
Step 4: Create View File
In this step, we will make the view file imageUploadForm.php in the view folder of CodeIgniter 3. Using the form helper and the url helper, we will create an HTML form design in this file. Therefore, let's update this file:
application/views/imageUploadForm.php
<!DOCTYPE html>
<html>
<head>
<title>Codeignier 3 Image Upload with Resize Example from Scratch</title>
</head>
<body>
<?php echo $error;?>
<?php echo form_open_multipart('image-upload/post');?>
<input type="file" name="image" size="20" />
<input type="submit" value="upload" />
</form>
</body>
</html>
that's all, we are ready to roll now.
Two folders need to be created now. First, you must create the "uploads" folder, and then you must create the "thumbnail" folder within the uploads folder.
You will see a thumbnail image in the thumbnail directory after running.
Let's run and see.
Hope this post will help you to resize an image and make a thumbnail of that image using CodeIgniter 3
What's Your Reaction?
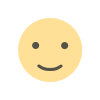
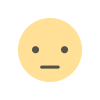
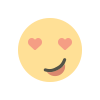
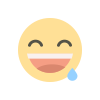
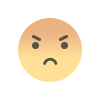
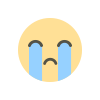
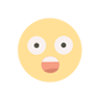