What is JSON data ? JSON data communication with different programming languages
JSON data is a lightweight format for storing and exchanging data. It stands for JavaScript Object Notation and is easy for humans to read and write, and for machines to parse and generate. JSON is commonly used in web applications to send data between the server and the client.
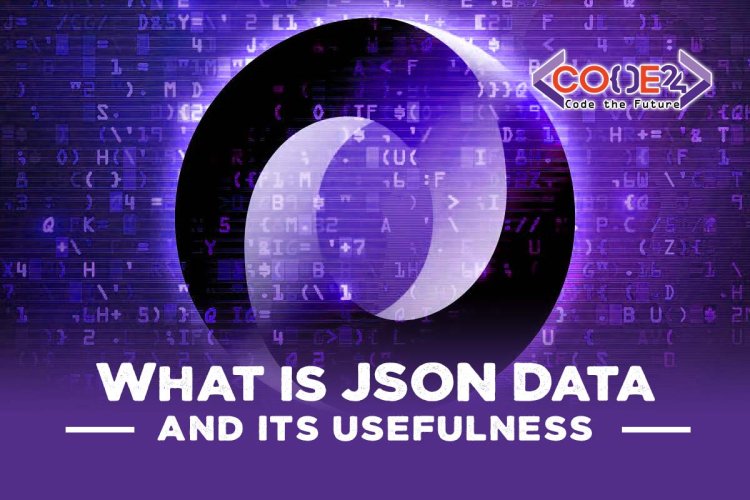
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format used to store and transfer data. It has become a popular choice for data storage and exchange due to its simplicity and ease of use. This blog will explore what JSON data is, why it's important, and how to use it effectively.
What is JSON Data?
JSON data is a text-based format that is used to represent data objects as a collection of key-value pairs. It is based on a subset of the JavaScript programming language and is designed to be both human-readable and machine-readable. JSON data is often used in web applications to transfer data between servers and clients.
Why is JSON Data Important?
JSON data is important because it provides a standardized way to transfer data between applications. It is lightweight, easy to read, and can be parsed by a wide variety of programming languages. JSON data is also easily integrated with JavaScript, which is commonly used in web development.
What does JSON data look like?
JSON data consists of a collection of name/value pairs, where the name is a string and the value can be a string, number, boolean, array, or another JSON object. Here's an JSON data example of what JSON data might look like:
{
"name": "John",
"age": 30,
"isEmployed": true,
"favoriteColors": ["blue", "green", "yellow"],
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345"
}
}
In this example, the JSON data represents information about a person, including their name, age, employment status, favorite colors, and address. The name/value pairs are separated by commas, and the entire data structure is enclosed in curly braces.
The "name", "age", and "isEmployed" properties are all strings or booleans, while the "favoriteColors" property is an array of strings. The "address" property is another JSON object that contains multiple name/value pairs.
JSON data can be more complex than this example, with multiple nested objects and arrays, but the basic structure is always the same: a collection of name/value pairs that can be easily parsed and manipulated by software applications.
How to use JSON Data Effectively?
When using JSON data, it's important to follow best practices to ensure that your data is easy to read and parse. Here are some tips for using JSON data effectively:
- Use Consistent Formatting: Use consistent formatting for your JSON data to make it easier to read and parse. This includes using indentation and line breaks to organize your data.
- Use Descriptive Key Names: Use descriptive key names that accurately describe the data they represent. This makes it easier for developers to understand and use your data.
- Avoid Using Nested Objects: Avoid using nested objects in your JSON data, as they can be difficult to parse and can lead to performance issues.
- Use Arrays for Lists of Data: Use arrays to represent lists of data in your JSON data. This makes it easy to iterate over the list and extract individual items.
- Validate Your JSON Data: Use a JSON validator to ensure that your data is valid and properly formatted. This can help you avoid errors and improve the performance of your applications.
How to use JSON data with some common programming language?
JSON data can be used with a wide variety of programming languages. Here are some examples of how to use JSON data with some common programming languages:
1. JavaScript:
JSON is based on a subset of the JavaScript programming language, so using JSON in JavaScript is very straightforward. You can use the built-in JSON object to parse and stringify JSON data. Here is an example:
// Parsing JSON data
const jsonData = '{"name": "John", "age": 30}';
const obj = JSON.parse(jsonData);
console.log(obj.name); // Output: John
// Stringifying JavaScript object to JSON
const person = {name: "John", age: 30};
const jsonString = JSON.stringify(person);
console.log(jsonString); // Output: {"name":"John","age":30}
2. Python:
Python provides a built-in module called json for working with JSON data. You can use the json.loads() function to parse JSON data and json.dumps() function to convert Python objects to JSON. Here is an example:
import json
# Parsing JSON data
json_string = '{"name": "John", "age": 30}'
obj = json.loads(json_string)
print(obj["name"]) # Output: John
# Converting Python object to JSON
person = {"name": "John", "age": 30}
json_string = json.dumps(person)
print(json_string) # Output: {"name": "John", "age": 30}
3. Java:
Java provides a library called json-simple for working with JSON data. You can use the JSONParser class to parse JSON data and the JSONObject and JSONArray classes to create and manipulate JSON objects. Here is an example:
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
// Parsing JSON data
String json_string = "{\"name\": \"John\", \"age\": 30}";
JSONParser parser = new JSONParser();
JSONObject obj = (JSONObject) parser.parse(json_string);
System.out.println(obj.get("name")); // Output: John
// Creating JSON object
JSONObject person = new JSONObject();
person.put("name", "John");
person.put("age", 30);
String json_string = person.toJSONString();
System.out.println(json_string); // Output: {"name":"John","age":30}
4. PHP:
PHP provides built-in functions for working with JSON data, such as json_decode() and json_encode(). Here is an example:
// Parsing JSON data
$json_string = '{"name": "John", "age": 30}';
$obj = json_decode($json_string);
echo $obj->name; // Output: John
// Creating JSON object
$person = new stdClass();
$person->name = "John";
$person->age = 30;
$json_string = json_encode($person);
echo $json_string; // Output: {"name":"John","age":30}
Why does API commonly use JSON data to communicate?
APIs (Application Programming Interfaces) are used to facilitate communication between software applications. They allow developers to access data and functionality from other applications, often over the internet. JSON data is a popular choice for API communication for several reasons:
- Lightweight: JSON is a lightweight data format that is easy to transfer over the internet. This makes it an ideal choice for APIs, which often involve large amounts of data being transferred between applications.
- Human-readable: JSON data is easy for humans to read and understand, which makes it easier for developers to work with. This can help speed up the development process and reduce the likelihood of errors.
- Widely supported: JSON is widely supported across many different programming languages, making it easy for developers to work with regardless of their platform or language of choice.
- Easy to parse: JSON data is easy to parse and manipulate using programming languages. This makes it easy for developers to extract the data they need from API responses and use it in their applications.
- Compatible with JavaScript: JSON is based on a subset of the JavaScript programming language, which makes it easy to use in web applications that use JavaScript.
Overall, JSON data is a popular choice for API communication because of its simplicity, readability, and wide support across different programming languages
Conclusion
JSON data is a powerful tool for storing and transferring data between applications. It is lightweight, easy to read, and can be parsed by a wide variety of programming languages. By following best practices for using JSON data, you can ensure that your data is easy to work with and helps your applications run smoothly.
What's Your Reaction?
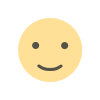
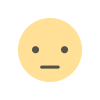
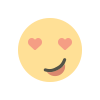
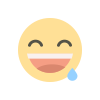
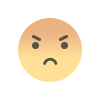
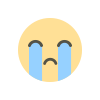
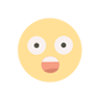